Intro to jQuery
JavaScript made more accessible
Created by Brian Duffey
Who am I?
- Brian Duffey
-
4 years consultant at michaels, ross, and cole
- 4+ years jQuery developer
- What have I used jQuery for?
Where are we going?
- Introduction
- Who am I?
- Where are we going?
- What is jQuery?
- Why was it created?
- Why would you use it?
- Adding jQuery to Your Pages
- Should you host or include?
- How do you bring the library into your pages?
- How can you write your own scripts?
- Using jQuery on Your Pages
- What are selectors?
- What do you do with selected elements?
- Where do you go from here?
Where are we going?
- Introduction
- Who am I?
- Where are we going?
- What is jQuery?
- Why was it created?
- Why would you use it?
- Adding jQuery to Your Pages
- Should you host or include?
- How do you bring the library into your pages?
- How can you write your own scripts?
- Using jQuery on Your Pages
- What are selectors?
- What do you do with selected elements?
- Where do you go from here?
What is 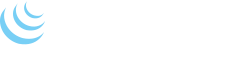
- Free, open source library
- Written over JavaScript
- Created by John Resig
- Initially released in 2006
Why was it created?
All Sites |
|
|
Top 1000 |
|
|
Top 100 |
|
|
-from http archive, http://httparchive.org/interesting.php
Why was it created?
JavaScript
- Very useful in modern web development
- Can be hard to learn
- Long scripts for simple functions cause readability issues
jQuery
- Simplifies existing JavaScript functions
- Easy to learn as it uses existing CSS selectors
- Simpler to read and understand as less code is required
Why was it created?
JavaScript Demo
window.onload = function () {
var myName, greeting, elems;
myName = prompt('What is your name?');
greeting = document.getElementById('myName');
str = 'Hello, ' + myName;
greeting.innerHTML = str;
elems = document.getElementsByTagName('input');
for (var elem in elems) {
if (elems[elem].type === 'button') {
elems[elem].addEventListener('click', function () {
changeColor(this.value, 'box')
}, false);
}
}
};
function changeColor(color, el) {
var elems2 = document.getElementsByTagName('div');
for (var i = 0, j = elems2.length; i < j; i++) {
if ((' ' + elems2[i].className + ' ').indexOf(' ' + el + ' ') > -1) {
elems2[i].style.background = color;
}
}
}
Why was it created?
jQuery Demo
jQuery(function () {
jQuery('#myName').text('Hello, ' + prompt('What is your name?'));
jQuery('input[type="button"]').click(function () {
changeColor(jQuery(this).val(), 'box');
});
});
function changeColor(color, el) {
jQuery('.' + el).css('background', color);
}
Why would you use jQuery?
- jQuery was the first JS library to be fully documented
- There are numerous plugins available for whatever you need
- jQuery has a very small footprint in your page - 32kB
- jQuery fails gracefully when selectors not found
- jQuery has wide support for browsers
- IE6+
- Chrome
- Firefox
- Safari
- Opera
- Mobile - iOS/Android
-
-from W3Techs, http://w3techs.com/technologies/details/js-jquery/all/all
Where are we going?
- Introduction
- Who am I?
- Where are we going?
- What is jQuery?
- Why was it created?
- Why would you use it?
- Adding jQuery to Your Pages
- Should you host or include?
- How do you bring the library into your pages?
- How can you write your own scripts?
- Using jQuery on Your Pages
- What are selectors?
- What do you do with selected elements?
- Where do you go from here?
Should you host or include?
Hosting
...involves downloading the jQuery library to your server, and including the library from there
- Why you might:
- More secure
- More reliable
Should you host or include?
Including
...involves linking to the jQuery library from a CDN (Content Delivery Network)
- Why you might:
- Decreased latency
- Increased parallelism
- Better caching
Where to get it?
Hosting
Download latest version from jQuery.com
Including
There are many trusted CDNs
- Google
- jQuery.com
- Microsoft
How do you include the library in your pages?
Use script tags, just like any other JavaScript file:
Or:
Where to include jQuery on your pages?
Unlike CSS, keep all scripts at bottom of page
Why?
- HTML Pages load from top to bottom
- Load styles as early as possible
- Delay loading of scripts until necessary
<!DOCTYPE HTML>
<html>
<head>
Title
</head>
<body>
Your content
</body>
</html>
How can you include your own scripts?
Either include them inline...
...or bring them in from an external file just like jQuery
How can you include your own scripts?
If bringing in/writing scripts at the top of your page, use jQuery(document).ready():
jQuery(document).ready(function() { /* your script in here */ });
This can also be shortened:
jQuery(function() { /* your script in here */ });
This allows the page to load fully before executing your script
Where are we going?
- Introduction
- Who am I?
- Where are we going?
- What is jQuery?
- Why was it created?
- Why would you use it?
- Adding jQuery to Your Pages
- Should you host or include?
- How do you bring the library into your pages?
- How can you write your own scripts?
- Using jQuery on Your Pages
- What are selectors?
- What do you do with selected elements?
- Where do you go from here?
What are selectors?
- Based on CSS selectors
- Used to select elements from the DOM
- Returns zero, one, or more objects that match
- Takes the form: jQuery('div')
What are selectors?
jQuery('div')
- Calls the jQuery library
- Commonly written as $
- Avoid conflicts with other libraries by using jQuery
What are selectors?
jQuery('div')
- Selects some element
- jQuery reads string value to determine element
- Can be a literal (like 'div') or variable (like someElement)
What are selectors?
Single Selectors
- Element:
Selector | Element |
div | ..
|
p | ..
|
span | ..
|
- Class:
Selector | Element |
.someClass | ..
|
- ID:
Selector | Element |
#someID | ..
|
What are selectors?
Multiple Selectors
What are selectors?
Traversing the DOM
- Good markup will allow you to easily select an object or objects
- Sometimes this is not possible
- Instead, you can "walk the DOM" to find the object
- This is done with various jQuery functions, such as:
- .parent()
- .children()
- .find()
- .filter()
- .after()
- .before()
What are selectors?
Chaining
- Every jQuery function that doesn't return some value, returns the current object
- This allows for "chaining" or performing different actions on the same object(s)
- This greatly shortens code:
jQuery('div').css('color', 'red');
jQuery('div').css('backgroundColor', 'blue');
jQuery('div').css('color', 'red').css('backgroundColor', 'blue');
What are selectors?
Putting it all together
- Often, selectors are 80% of jQuery
- Sometimes your element will have an ID and be simple to select
- Other times you will have to walk the DOM a bit to select it
- Here is a good visual of various selectors
OK, so now what?
We've selected the heck out of our elements
Now it's time to do something with them
In general, the four areas you might focus on are:
- Modifying - changing some element
- Manipulating - changing the page around some element
- Listening - responding to events on some element
- Animating - Moving or transforming some element
Modifying
Involves changing the selected element(s) in some way
Manipulating
Involves changing the DOM directly
- .append()/.prepend()
- .clone()
- .load()
Manipulating
.clone()
- Allows you to copy the selected element(s)
jQuery('div').clone();
jQuery('p').clone(true);
Demo
Listening
Involves calling some code after some event on the selected element(s) happens
- .click()
- .hover()
- .change()
Animating
Involves moving/redrawing the element(s) on the page
- .hide()/.show()/.toggle()
- .slide()
- .fade()
Where are we going?
- Introduction
- Who am I?
- Where are we going?
- What is jQuery?
- Why was it created?
- Why would you use it?
- Adding jQuery to Your Pages
- Should you host or include?
- How do you bring the library into your pages?
- How can you write your own scripts?
- Using jQuery on Your Pages
- What are selectors?
- What do you do with selected elements?
- Where do you go from here?
Links
- My information:
- Slides:
- Webinars:
- Other resources:
- jsFiddle
- jQuery.com
- StackOverflow